ADB over Wi-Fi
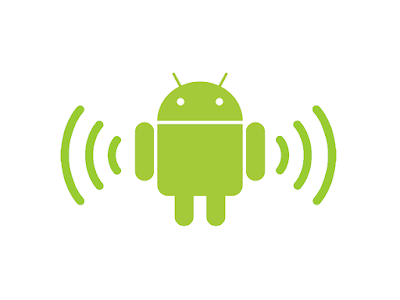
To debug the behavior of your application while an accessory is plugged in, you can't use ADB through a USB cable. In this case, to debug applications, you need to link your device to ADB over Wi-Fi. Steps to configure the ADB connection over Wi-Fi Connect the device and the computer to the same Wi-Fi network. If the computer is connected to VPN, disconnect from it. The device can be on VPN. Plug the device into the computer with a USB cable to configure the connection On the computer command line type: adb tcpip 5555 On the computer command line type: adb shell ip addr show wlan0 and copy the IP address after the "inet" until the "/". You can also go inside the Settings of the device to retrieve the IP address in Settings → About → Status. On the computer command line type: adb connect ip-address-of-device:5555 You can disconnect the USB cable from the device and check with adb devices that the device is still detected. adb over wifi